Object Oriented Programming in PHP -3 [The $this keyword]
What is $this Keyword
In PHP, $this
keyword references the current object of the class. The $this
keyword allows you to access the properties and methods of the current object within the class using the object operator (->
):
$this->property
$this->method()
How $this Keyword Works
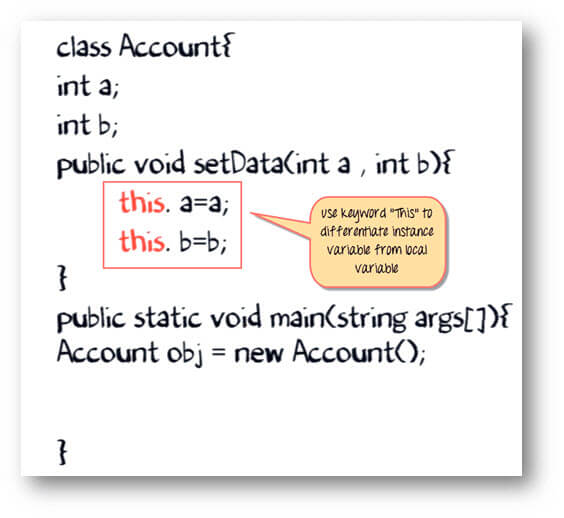
During code execution when an object calls the method ‘setdata’. The keyword ‘this’ is replaced by the object handler “obj.” (See the image below).

Scope of $this Keyword
The
keyword is only available within a class. It doesn’t exist outside of the class. If you attempt to use the $this
outside of a class, you’ll get an error.$this
Rules to use $this Keyword
When you access an object property using the
keyword, you use the $this
$
with the this
keyword only. And you don’t use the $
with the property name. For example:
$this->balance
The following shows the BankAccount
class:
<?php class BankAccount { public $accountNumber; public $balance; public function deposit($amount) { if ($amount > 0) { $this->balance += $amount; } } public function withdraw($amount) { if ($amount <= $this->balance) { $this->balance -= $amount; return true; } return false; } }
In the above example, we access the balance
property via the $this
keyword inside the deposit()
and withdraw()
methods.
In the first tutorial of this series, we learned how to group the code related to a certain topic into one single class. As an example, we wrote a Car class that groups the code that handles cars.
class Car {
public $comp;
public $color = 'beige';
public $hasSunRoof = true;
public function hello()
{
return "beep";
}
}
We also created two objects out of the class in order to be able to use its code by those two objects.
$bmw = new Car ();
$mercedes = new Car ();
The $this keyword
The $this keyword indicates that we use the class’s own methods and properties, and allows us to have access to them within the class’s scope.
The $this keyword allows us to approach the class properties and methods from within the class using the following syntax:
Only the $this keyword starts with the $ sign, while the names of the properties and methods do not start with it.
$this -> propertyName;
$this -> methodName();
Let’s illustrate what we have just said on the Car class. We will enable the hello() method to approach the class’s own properties by using the $this keyword.
In order to approach the class $comp property. We use:
$this -> comp
In order to approach the class $color property. We use:
$this -> color
That’s what the code looks like:
class Car {
// The properties
public $comp;
public $color = 'beige';
public $hasSunRoof = true;
// The method can now approach the class properties
// with the $this keyword
public function hello()
{
return "Beep I am a <i>" . $this -> comp . "</i>, and I am <i>" .
$this -> color;
}
}
Let us create two objects from the class:
$bmw = new Car();
$mercedes = new Car ();
and set the values for the class properties:
$bmw -> comp = "BMW";
$bmw -> color = "blue";
$mercedes -> comp = "Mercedes Benz";
$mercedes -> color = "green";
We can now call the hello() method for the first car object:
echo $bmw -> hello();
Result:
And for the second car object.
echo $mercedes -> hello();
Result:
Beep I am a Mercedes Benz, and I am green.
This is the code that we have written in this tutorial:
class Car {
// The properties
public $comp;
public $color = 'beige';
public $hasSunRoof = true;
// The method that says hello
public function hello()
{
return "Beep I am a <i>" . $this -> comp .
"</i>, and I am <i>" . $this -> color;
}
}
// We can now create an object from the class.
$bmw = new Car();
$mercedes = new Car();
// Set the values of the class properties.
$bmw -> color = 'blue';
$bmw -> comp = "BMW";
$mercedes -> comp = "Mercedes Benz";
// Call the hello method for the $bmw object.
echo $bmw -> hello();